Spring Mvc File Upload With Java Configuration
Jump MVC provides out of box back up for multiple file upload functionality in any application. This tutorial uses CommonsMultipartResolver
and requires apache commons fileupload and apache commons io dependencies.
<dependency> <groupId>commons-fileupload</groupId> <artifactId>eatables-fileupload</artifactId> <version>1.three.ane</version> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>two.6</version> </dependency>
1. Bound MVC MultipartFile Interface
A file uploaded to a Spring MVC application will be wrapped in a MultipartFile object. All we demand to practise is to write a domain class with a property of type MultipartFile
. This interface has methods for getting the name and content of an uploaded file e.one thousand. getBytes()
, getInputStream()
, getOriginalFilename()
, getSize()
, isEmpty()
and tranferTo()
.
For example, to salvage the uploaded file to file organisation, we can use the transferTo method:
File file = new File(...); multipartFile.transferTo(file);
2. Domain course for file upload
You need to create a elementary domain course with necessary attributes and one for storing files of type Listing<MultipartFile>
.
To build this example, I accept written this domain grade.
public class Product implements Serializable { individual static final long serialVersionUID = 74458L; @NotNull @Size(min=ane, max=10) private String name; private String description; individual Listing<MultipartFile> images; //getters and setters }
three. Spring MVC multi-file upload controller
In controller grade, nosotros will get pre-populated details of uploaded files in domain class. Only fetch the details and store the files in file system or database every bit per application pattern.
@Controller public class DemoProductController { @RequestMapping("/salvage-product") public Cord uploadResources( HttpServletRequest servletRequest, @ModelAttribute Product product, Model model) { //Go the uploaded files and store them List<MultipartFile> files = production.getImages(); List<String> fileNames = new ArrayList<String>(); if (null != files && files.size() > 0) { for (MultipartFile multipartFile : files) { Cord fileName = multipartFile.getOriginalFilename(); fileNames.add(fileName); File imageFile = new File(servletRequest.getServletContext().getRealPath("/image"), fileName); try { multipartFile.transferTo(imageFile); } catch (IOException e) { due east.printStackTrace(); } } } // Hither, yous can salve the production details in database model.addAttribute("production", product); return "viewProductDetail"; } @RequestMapping(value = "/product-input-form") public String inputProduct(Model model) { model.addAttribute("production", new Product()); return "productForm"; } }
iv. Spring MVC configuration changes
To support multipart request, we will need to declare multipartResolver bean in configuration file.
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> <property name="maxUploadSize" value="20848820" /> </edible bean>
Equivalent Coffee note configuration is :
@Bean(name = "multipartResolver") public CommonsMultipartResolver multipartResolver() { CommonsMultipartResolver multipartResolver = new CommonsMultipartResolver(); multipartResolver.setMaxUploadSize(20848820); return multipartResolver; }
Additionally, we may want to map the file storage path on server as resource. This will be bound mvc file upload directory.
<mvc:resource mapping="/image/**" location="/prototype/" />
The consummate configuration file used for this example is:
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://world wide web.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-iii.0.xsd http://world wide web.springframework.org/schema/context/ http://www.springframework.org/schema/context/leap-context-iii.0.xsd http://world wide web.springframework.org/schema/mvc/ http://www.springframework.org/schema/mvc/spring-mvc-three.0.xsd"> <context:component-browse base of operations-parcel="com.howtodoinjava.demo" /> <mvc:resources mapping="/epitome/**" location="/image/" /> <edible bean form="org.springframework.web.servlet.mvc.annotation.AnnotationMethodHandlerAdapter" /> <bean class="org.springframework.web.servlet.mvc.annotation.DefaultAnnotationHandlerMapping" /> <edible bean class="org.springframework.spider web.servlet.view.InternalResourceViewResolver"> <property proper noun="prefix" value="/WEB-INF/views/" /> <property name="suffix" value=".jsp" /> </bean> <edible bean id="messageSource" grade="org.springframework.context.back up.ResourceBundleMessageSource"> <property name="basename" value="messages" /> </bean> <bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> <belongings name="maxUploadSize" value="20848820" /> </bean> </beans>
5. Spring MVC view to upload files
I have written ii JSP files. One for showing the file upload form where user will fill the other details and choose files to upload. Second where we will display the upload files with other details.
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> <!DOCTYPE html> <html> <head> <championship>Add together Product Class</title> </caput> <trunk> <div id="global"> <class:form commandName="product" activeness="save-product" method="mail" enctype="multipart/grade-data"> <fieldset> <fable>Add together a product</fable> <p> <label for="name">Product Name: </characterization> <form:input id="name" path="name" cssErrorClass="error" /> <grade:errors path="name" cssClass="error" /> </p> <p> <characterization for="description">Description: </label> <form:input id="clarification" path="description" /> </p> <p> <label for="image">Product Images: </label> <input blazon="file" name="images" multiple="multiple"/> </p> <p id="buttons"> <input id="reset" blazon="reset" tabindex="four"> <input id="submit" blazon="submit" tabindex="v" value="Add Product"> </p> </fieldset> </class:form> </div> </body> </html>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <!DOCTYPE html> <html> <head> <championship>Relieve Product</championship> </head> <body> <div id="global"> <h4>The product has been saved.</h4> <h5>Details:</h5> Product Name: ${product.name}<br/> Description: ${product.description}<br/> <p>Following files are uploaded successfully.</p> <ol> <c:forEach items="${product.images}" var="image"> <li>${image.originalFilename} <img width="100" src="<c:url value="/image/"/>${image.originalFilename}"/> </li> </c:forEach> </ol> </div> </torso> </html>
Spring MVC multiple file upload case
When we hitting the browser with http://localhost:8080/springmvcexample/product-input-grade
, nosotros get following screen:
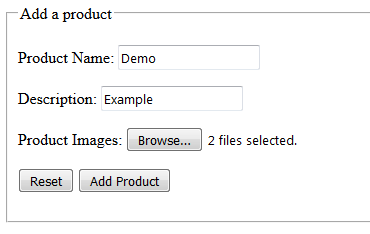
we fill the details and submit the class and we will get the submitted details and all uploaded files in other page:
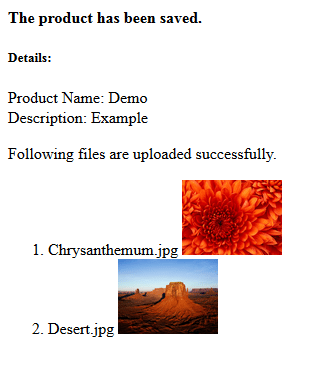
Drib me your questions and suggestions in comments section related to this spring mvc multipart file upload example.
Happy Learning !!
Source: https://howtodoinjava.com/spring-mvc/spring-mvc-multi-file-upload-example/
Postar um comentário for "Spring Mvc File Upload With Java Configuration"